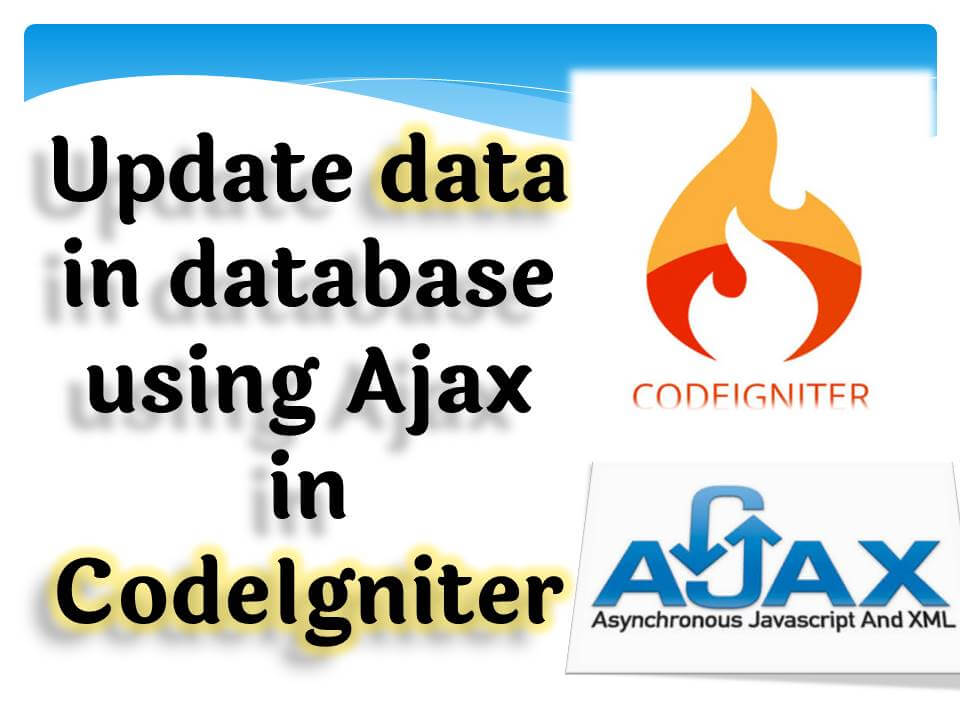
Ajax Codeigniter CRUD Tutorial के इस series में मैं आपको बताऊंगा की Database में Ajax और Codeigniter का इस्तेमाल करके data को कैसे update करें – How to update data in Codeigniter using Ajax?
इससे पहले के tutorial में मैंने बताया था की Ajax का इस्तेमाल करके Codeigniter में data को database में कैसे insert करें? और Datatable में Ajax का इस्तेमाल करके Data को कैसे show कराएं? अगर उस tutorial को आपने नहीं देखा और पढ़ा है तो सबसे पहले उसे पढ़ें तभी यह tutorial आपके समझ में आएगी|
Ajax का इस्तेमाल करके हम data को बहुत ही आसानी से और fast way में update कर सकते हैं इससे Browser page refresh नहीं होता है जिसके कारण बहुत fast work होता है| आज के दिन में हर website या social media platform without refreshing page पर काम कर रही है जिससे user experience अच्छा हो और उनका काम भी fast हो|
Update Data in Codeigniter using Ajax (Hindi Tutorial)
इससे पहले के tutorial में मैंने Table create करने का code add कर दिया है| इस tutorial में आपको Controller, Views, और Model में कुछ extra code add करने हैं जो की निचे बताया गया है|
Controller – MY_Controller.php
Controller में मैंने दो नए functions को add किया है जिनके नाम इस प्रकार हैं: getEditData() और update(). getEditData() function में मैं database से एक particular id का data fetch कर रहा हूँ जिस data को हमें edit करना है| और update() function में मैं data को update कर रहा हूँ|
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class MY_Controller extends CI_Controller {
public function __construct()
{
parent::__construct();
$this->load->model('ajax_model');
}
public function index()
{
$this->load->view('index');
}
public function create()
{
$name = $this->input->post('name');
$message = $this->input->post('message');
$age = $this->input->post('age');
$data = array(
'name' => $name,
'message' => $message,
'age' => $age,
);
$insert = $this->ajax_model->createData($data);
echo json_encode($insert);
}
public function fetchDatafromDatabase()
{
$resultList = $this->ajax_model->fetchAllData('*','person',array());
$result = array();
$button = '';
$i = 1;
foreach ($resultList as $key => $value) {
$button = '<a class="btn-sm btn-success text-light" onclick="editFun('.$value['id'].')" href="#"> Edit</a>';
$result['data'][] = array(
$i++,
$value['name'],
$value['message'],
$value['age'],
$button
);
}
echo json_encode($result);
}
public function getEditData()
{
$id = $this->input->post('id');
$resultData = $this->ajax_model->fetchSingleData('*','person',array('id'=>$id));
echo json_encode($resultData);
}
public function update()
{
$id = $this->input->post('id');
$name = $this->input->post('name');
$message = $this->input->post('message');
$age = $this->input->post('age');
$data = array(
'name' => $name,
'message' => $message,
'age' => $age,
);
$update = $this->ajax_model->updateData('person',$data,array('id'=>$id));
if($update==true)
{
echo 1;
}
else{
echo 2;
}
}
}
Model – Ajax_Model.php
Model में मैंने दो नए functions को add किया है जिनके नाम क्रमशः इस प्रकार हैं: fetchSingleData() और updateData(). fetchSingleData() function में हम किसी particular row को fetch करते हैं जबकि updateData() function के द्वारा हम database में data को update करने का काम करते हैं|
<?php
class Ajax_model extends CI_Model
{
public function createData($data)
{
$query = $this->db->insert('person',$data);
return $query;
}
public function fetchAllData($data,$tablename,$where)
{
$query = $this->db->select($data)
->from($tablename)
->where($where)
->get();
return $query->result_array();
}
public function fetchSingleData($data,$tablename,$where)
{
$query = $this->db->select($data)
->from($tablename)
->where($where)
->get();
return $query->row_array();
}
public function updateData($tablename, $data, $where)
{
$query = $this->db->update($tablename,$data,$where);
return $query;
}
public function deleteData($tablename,$where)
{
$query = $this->db->delete($tablename,$where);
return $query;
}
public function insertDynamicData($tablename, $data)
{
$query = $this->db->insert($tablename, $data);
return $query;
}
}
Views – index.php
Views में मैंने एक Bootstrap modal add किया है जिसका id editModal है और उसके कुछ fields में मैंने ID set किये हैं| JavaScript में एक function बनाया है जिसका नाम editFun(id) है और इसमें id नाम का एक variable के द्वारा parameter से पास किये गए id का value को accept कर रहा हूँ| उसके बाद Ajax के द्वारा controller को कॉल करके उस particular id का data fetch कर रहा हूँ जिसे हमें edit करना है|
उसके बाद Finally Form submit के लिए हमने कुछ code लिखें है जिसका इस्तेमाल करके हम database में data को update कर रहे हैं|
<!DOCTYPE html>
<html>
<head>
<title></title>
<link rel="stylesheet" type="text/css" href="https://cdn.datatables.net/1.10.19/css/jquery.dataTables.min.css">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<br><br>
<!-- Button trigger modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#createModal">
Add Record
</button><br><br>
<form id="createForm">
<!-- Modal -->
<div class="modal fade" id="createModal" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLongTitle">Add Record</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<div class="form-group">
<label>Name</label>
<input type="text" class="form-control" placeholder="Name here" name="name">
</div>
<div class="form-group">
<label>Message</label>
<input type="text" class="form-control" placeholder="Message Here" name="message">
</div>
<div class="form-group">
<label>Age</label>
<input type="number" class="form-control" placeholder="Age Here" name="age">
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary pull-left" data-dismiss="modal">Close</button>
<button type="submit" class="btn btn-primary">Save</button>
</div>
</div>
</div>
</div>
</form>
<table id="example1" class="display table">
<thead>
<tr>
<th>S.No</th>
<th>Name</th>
<th>Message</th>
<th>Age</th>
<th>Action</th>
</tr>
</thead>
</table>
</div>
<!-- edit modal -->
<div class="modal fade" id="editModal" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">Edit Record</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<form id="editForm">
<div class="modal-body">
<input type="hidden" name="id" id="editID">
<div class="form-group">
<label>Name</label>
<input type="text" class="form-control" placeholder="Name here" name="name" id="editName">
</div>
<div class="form-group">
<label>Message</label>
<input type="text" class="form-control" placeholder="Message Here" name="message" id="editMessage">
</div>
<div class="form-group">
<label>Age</label>
<input type="number" class="form-control" placeholder="Age Here" name="age" id="editAge">
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary pull-left" data-dismiss="modal">Close</button>
<button type="submit" class="btn btn-primary">Update</button>
</div>
</form>
</div>
</div>
</div>
<!--edit modal end-->
<script type="text/javascript" src="https://code.jquery.com/jquery-3.3.1.min.js"></script>
<script type="text/javascript" src="https://cdn.datatables.net/1.10.19/js/jquery.dataTables.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script>
<script type="text/javascript">
//inserting data into database code start here
$("#createForm").submit(function(event) {
event.preventDefault();
$.ajax({
url: "<?php echo base_url('my_controller/create'); ?>",
data: $("#createForm").serialize(),
type: "post",
async: false,
dataType: 'json',
success: function(response){
$('#createModal').modal('hide');
$('#createForm')[0].reset();
alert('Successfully inserted');
$('#example1').DataTable().ajax.reload();
},
error: function()
{
alert("error");
}
});
});
//inserting data into database code end here
//displaying data on page start here
$(document).ready(function(){
$('#example1').DataTable({
"ajax" : "<?php echo base_url('my_controller/fetchDatafromDatabase'); ?>",
"order": [],
});
});
//displaying data on page end here
//edit function start here
function editFun(id)
{
$.ajax({
url: "<?php echo base_url('my_controller/getEditData'); ?>",
method:"post",
data:{id:id},
dataType:"json",
success:function(response)
{
$('#editID').val(response.id);
$('#editName').val(response.name);
$('#editMessage').val(response.message);
$('#editAge').val(response.age);
$('#editModal').modal({
backdrop:"static",
keyboard:false
});
}
})
}
$("#editForm").submit(function(event) {
event.preventDefault();
$.ajax({
url: "<?php echo base_url('my_controller/update'); ?>",
data: $("#editForm").serialize(),
type: "post",
async: false,
dataType: 'json',
success: function(response){
$('#editModal').modal('hide');
$('#editForm')[0].reset();
if(response==1)
{
alert('Successfully updated');
}
else{
alert('Updation Failed !');
}
$('#example1').DataTable().ajax.reload();
},
error: function()
{
alert("error");
}
});
});
//edit function work end here
</script>
</body>
</html>
इस tutorial को अच्छे से समझने के लिए Video Tutorial देखें|
Conclusion and Final Words
Ajax का इस्तेमाल करके बिना page को refresh किये आप data को update कर सकते हैं| इसके द्वारा काम बहुत ही fast होता है| तो आज एक इस tutorial में मैंने बताया की Ajax का इस्तेमाल करके Codeigniter में data कैसे update करें – Update data in codeigniter using Ajax?
मुझे उम्मीद है की यह tutorial आपके लिए काफी helpful रहा होगा| इसे अपने दोस्तों के साथ जरुर share करें| धन्यवाद|
model me bhi controller ka same code kr diya hai
model copy hai controler ka
change the your mistake
Thanks, Hitesh,
It will be updated soon